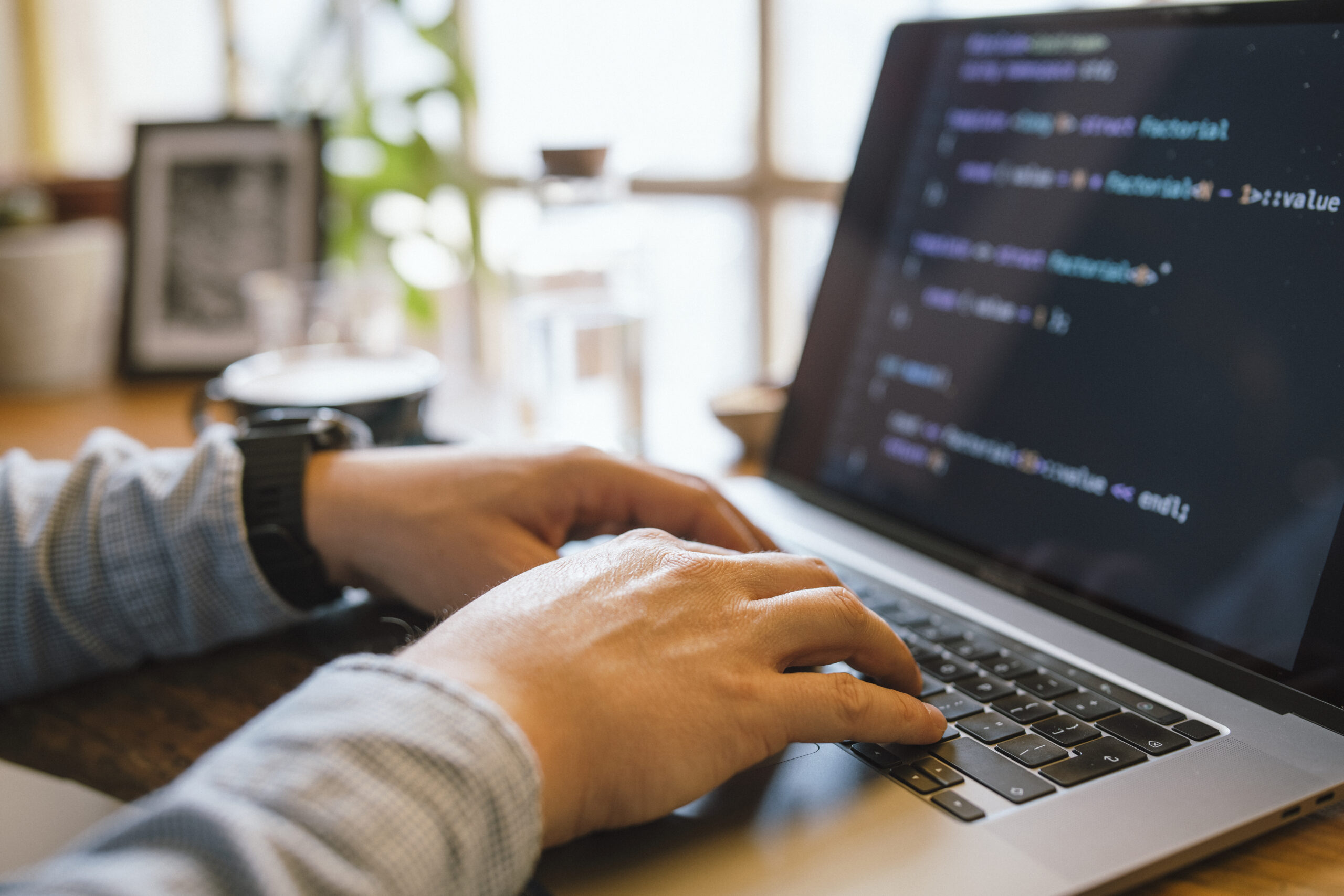
Debugging is Just about the most necessary — yet usually neglected — competencies inside of a developer’s toolkit. It's not just about fixing broken code; it’s about knowing how and why items go Mistaken, and Finding out to Assume methodically to unravel challenges successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Here's many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Portion of improvement, knowing ways to communicate with it successfully during execution is Similarly crucial. Modern day growth environments appear Outfitted with potent debugging abilities — but quite a few developers only scratch the area of what these resources can do.
Acquire, for example, an Built-in Growth Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools permit you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When used effectively, they let you notice just how your code behaves during execution, and that is priceless for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-finish builders. They let you inspect the DOM, monitor community requests, see authentic-time effectiveness metrics, and debug JavaScript while in the browser. Mastering the console, resources, and community tabs can change irritating UI difficulties into manageable duties.
For backend or process-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Studying these equipment can have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command methods like Git to comprehend code heritage, obtain the precise moment bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your resources implies heading over and above default configurations and shortcuts — it’s about acquiring an personal knowledge of your development atmosphere in order that when concerns come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote fixing the actual problem instead of fumbling via the process.
Reproduce the Problem
One of the most significant — and infrequently forgotten — methods in successful debugging is reproducing the trouble. Ahead of jumping into the code or earning guesses, builders need to have to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Check with queries like: What steps brought about the issue? Which ecosystem was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact disorders below which the bug takes place.
After you’ve collected more than enough details, try to recreate the challenge in your local setting. This may suggest inputting the same knowledge, simulating similar user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge conditions or state transitions included. These checks not just enable expose the issue and also stop regressions Sooner or later.
Sometimes, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or underneath particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It involves tolerance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you happen to be previously midway to repairing it. That has a reproducible state of affairs, You may use your debugging tools more successfully, test possible fixes safely, and communicate much more clearly together with your team or customers. It turns an abstract criticism right into a concrete obstacle — Which’s in which builders prosper.
Read through and Recognize the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders must find out to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it transpired, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in entire. Numerous builders, particularly when beneath time pressure, look at the very first line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just copy and paste mistake messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate triggered it? These inquiries can guideline your investigation and level you towards the responsible code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some mistakes are imprecise or generic, and in Individuals conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are not your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and become a much more effective and assured developer.
Use Logging Correctly
Logging is Among the most powerful tools inside a developer’s debugging toolkit. When utilised properly, it offers serious-time insights into how an application behaves, assisting you recognize what’s occurring underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic starts off with figuring out what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info throughout development, Facts for typical gatherings (like prosperous start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, condition changes, enter/output values, and important determination points in the code.
Structure your log messages Obviously and consistently. Incorporate context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it will require to identify problems, get further visibility into your applications, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a technical activity—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method just like a detective fixing a secret. This mentality helps break down complicated concerns into workable areas and abide by clues logically to uncover the foundation cause.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you could without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, form hypotheses. Request oneself: What could possibly be leading to this behavior? Have any changes recently been built to your codebase? Has this challenge transpired just before under similar instances? The target is usually to narrow down possibilities and detect likely culprits.
Then, examination your theories systematically. Attempt to recreate the condition in a very controlled environment. If you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code queries and let the effects direct you closer to the reality.
Pay out close awareness to tiny details. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for long run issues and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Write Tests
Composing assessments is among the simplest ways to boost your debugging techniques and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that offers you confidence when making modifications in your codebase. A perfectly-analyzed software is much easier to debug because it enables you to pinpoint precisely in which and when an issue occurs.
Start with unit tests, which concentrate on person functions or modules. These small, isolated tests can quickly expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and conclusion-to-conclude exams into your workflow. These help make sure a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in more info complex devices with several components or expert services interacting. If one thing breaks, your checks can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To check a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the exam fails regularly, you may focus on repairing the bug and enjoy your test pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return Down the road.
In brief, composing checks turns debugging from a aggravating guessing match right into a structured and predictable process—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—looking at your display for hrs, making an attempt Option immediately after Alternative. But one of the most underrated debugging tools is actually stepping away. Using breaks will help you reset your head, lessen annoyance, and infrequently see The problem from a new viewpoint.
When you are also close to the code for also extended, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or simply switching to a unique undertaking for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you face is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and evaluate what went Mistaken.
Start out by inquiring you a handful of key concerns once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots as part of your workflow or understanding and assist you to Establish stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring problems or common issues—you can proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily strong. No matter whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the same challenge boosts crew efficiency and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as essential portions of your improvement journey. In fact, a number of the best developers are usually not the ones who generate excellent code, but individuals that constantly master from their blunders.
Eventually, Every bug you deal with adds a fresh layer towards your skill established. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you happen to be knee-deep in a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.